队列的出现迎合了很多Java复杂结构的应用,如二叉树的层序遍历,本篇用两种方法(数组\链表)实现了队列的定义,与此同时还有一些经典的方法进行了定义,涵盖了简单队列的内容,欢迎阅览喔( ͡° ͜ʖ ͡°)✧
前言
与栈的结构相反,队列是一种先进先出的特殊表结构,普通队列的假溢出现象十分糟糕,所有目前对于队列的实现一般都使用循环队列,需要注意的是为了区分空队列和满队列的条件不同,故需要牺牲一个存储位置来满足循环队列的判断,具体可见数组形式的队列实现代码。
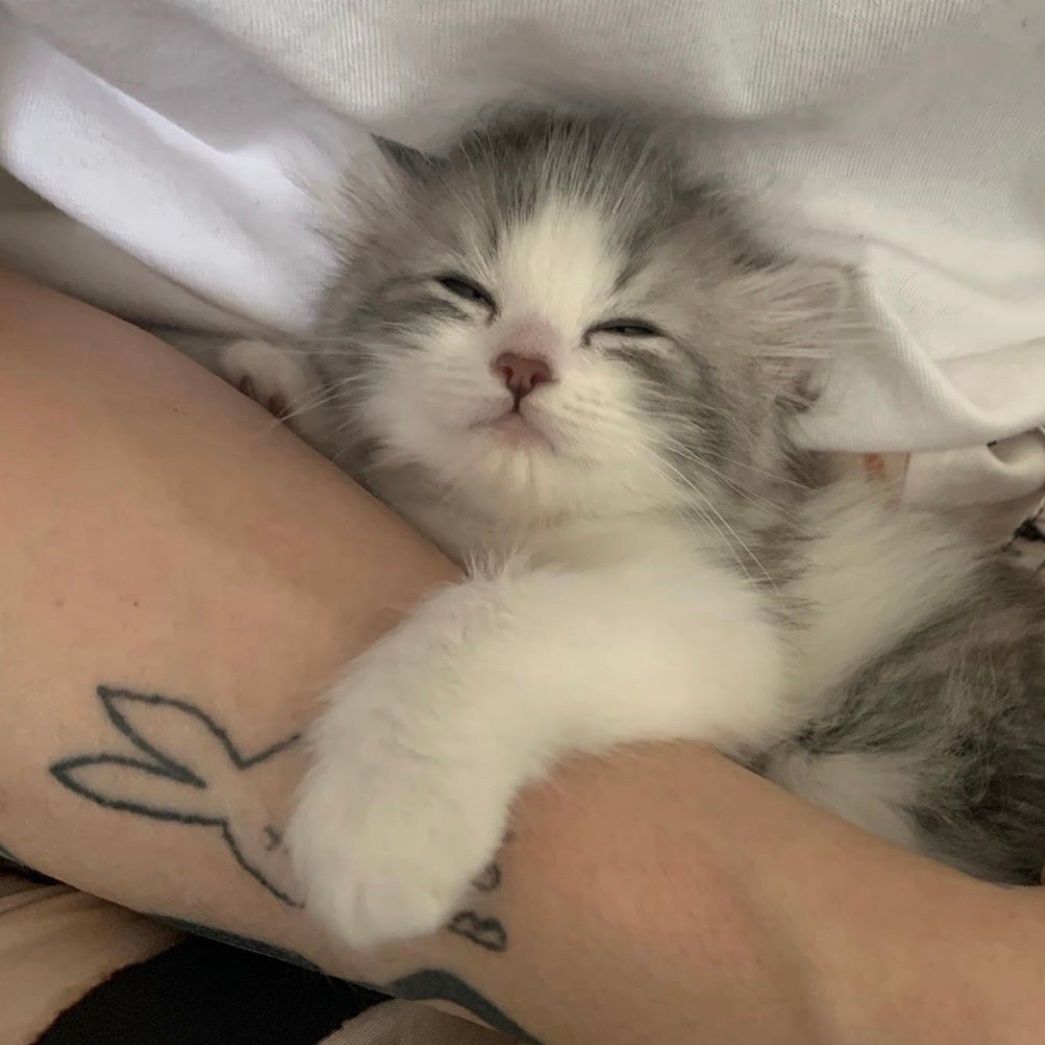
队列的数组实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102
| package SquenceList;
public class ArrayQueue<T> {
private T[] datas; private int rear; private int front; private int maxsize; private int realmaxsize; public ArrayQueue(int maxsize) { if(maxsize<1) { maxsize=1; } this.maxsize = maxsize; realmaxsize = maxsize-1; rear = 0; front = 0; datas = (T[])new Object[maxsize]; } public boolean isEmpty() { return (front==rear); } public boolean isFull() { if((rear+1)%maxsize==front) { rear--; return true; } return false; } public void push(T t) { if(isFull()) { System.out.println("队满啦,不能再进啦~"); return; } datas[rear]=t; rear++; rear = rear%maxsize; return; } public T pop() { if(isEmpty()) { System.out.println("我队什么都没有,不可以再出去了~"); return null; } T data = datas[front++]; front = front%maxsize; return data; } public int getFront() { return front; } public int getRear() { int end = rear-1; return end; } public T getFrontData() { return datas[front]; } public T getRearData() { int end = rear-1; return datas[end]; } public T[] getDatas() { return datas; } public int getLength() { return realmaxsize; } public static void main(String[] args) { ArrayQueue myqueue = new ArrayQueue(5); System.out.println("我的队列现在是空的吗:"+myqueue.isEmpty()); System.out.println("我的队列现在是满的吗:"+myqueue.isFull()); myqueue.push(1); System.out.println("现在队头是第几个呢:"+myqueue.getFront()); myqueue.push(3); myqueue.push(5); System.out.println("在我入队了一些元素后,现在我的队列现在是空的吗:"+myqueue.isEmpty()); System.out.println("在我入队了一些元素后,现在我的队列现在是满的吗:"+myqueue.isFull()); System.out.println("出队:"+myqueue.pop()); System.out.println("现在我的队伍都有谁呢:"+myqueue.getDatas()); System.out.println("现在队头是第几个呢:"+myqueue.getFront()); System.out.println("现在队尾是第几个呢:"+myqueue.getRear()); System.out.println("现在队头是谁呢:"+myqueue.getFrontData()); System.out.println("现在队尾是谁呢:"+myqueue.getRearData()); System.out.println("我的队列能放多少个元素呢:"+myqueue.getLength()); } }
|
队列的链表实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98
| package Queue;
public class LinkedQueue<T> {
private Node<T> front; private Node<T> rear; private int maxsize; class Node<T>{ private T data; public Node<T> next; public Node (){ this.data=null; this.next=null; } public Node(T data) { this.data = data; this.next = null; } public T getData() { return data; } public void setData(T data) { this.data = data; } public Node getNext() { return this.next; } } public LinkedQueue() { this.front = new Node(); this.rear = new Node(); maxsize = 0; } public void initQueue() { this.front = null; this.rear = null; maxsize = 0; } public boolean isEmpty() { if(front.next==null||rear.next==null) { return true; }else { return false; } } public void push(T data) { Node<T> node = new Node<T>(data); if(isEmpty()) { front.next = node; rear.next = node; maxsize++; }else { node.next = front.next; front.next = node; maxsize++; } } public Node pop() { if(isEmpty()) { System.out.println("这里是空的无法出队哦"); return null; }else if(maxsize ==1) { Node node = front.next; initQueue(); return node; }else { Node end = front; for(int i=1;i<maxsize-1;i++) { end = end.next; } Node node = rear.next; rear.next = end.next; maxsize--; return node; } } public static void main(String[] args) { LinkedQueue<Integer> lq = new LinkedQueue<Integer>(); System.out.println("我现在还没有入队,队是空的吗?"+lq.isEmpty()); lq.push(1); lq.push(2); lq.push(3); lq.push(4); System.out.println("我入队了一些元素,现在队还是空的吗?"+lq.isEmpty()); System.out.println("出队所有元素元素:"); System.out.println(lq.pop().data); System.out.println(lq.pop().data); System.out.println(lq.pop().data); System.out.println(lq.pop().data); } }
|